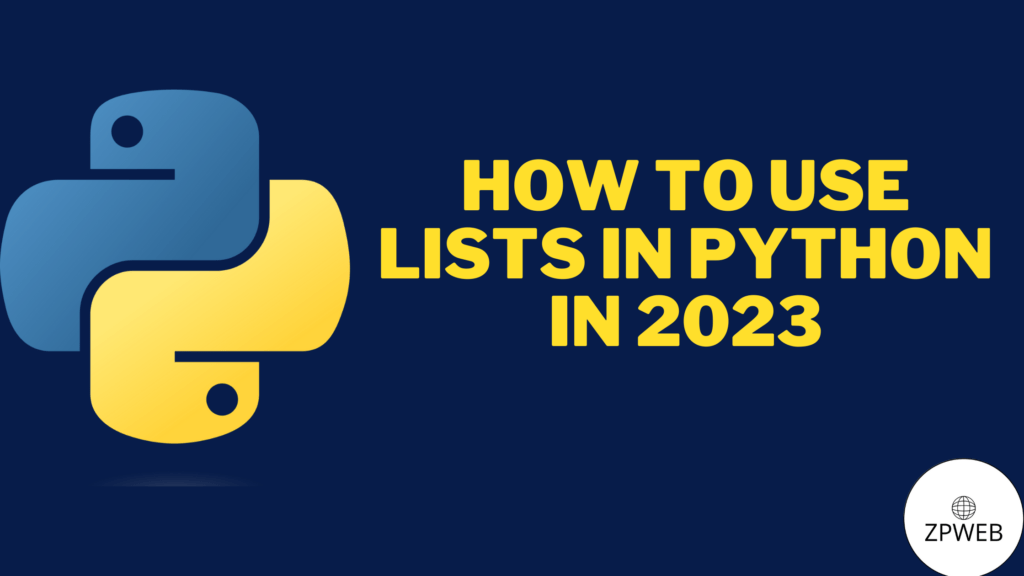
Lists in python are very useful if you want to store lots of data in one variable for example you want to get the usernames of people who sign up for a service and in this blog you will learn all about lists
A list in python can store any datatype including other lists that can be accessed by giving the right index
How to make a list
You can make list in python can be created by just by putting square brackets( ‘[]’ ) in the value for a variable for example:
my_list = []
How to write to a list
There are many ways to write to a list and I will show example on how to use all of them
Write the values when the list is being created
You can write values to the list when it is being created for example:
my_list = [1, 2, 3, 4]
Directly accessing the index
You can directly write values to a index like it is a variable but this only works if the index was already assigned to another value. You should use this when changing the value of the list
For example:
#this only works when the index you are on already has a value in it
my_list = [1,2,3,4]
#remember the index starts at 0 so for example the second element of a list would have an index of 0
my_list[0] = "Hello world"
print(my_list)
#Output
['Hello world', 2, 3, 4]
Appending the to the list
You can use the .append() command to put a value in the last index of the list. Use this command when the list is being used a lot
For example:
my_list = [1,2,3,4]
my_list.append(5)
print(my_list)
#Output
[1, 2, 3, 4, 5]
Inserting a value to the list
You can use the .insert() command to insert a value into the list by providing the index then the value. You should use this command when you know what order the value will have in the list
For example:
my_list = [2, 4, 6, 8]
my_list.insert(2, 90)
print(my_list)
#Output
[2, 4, 90, 8]
How to read from a list
There are two ways to read from a list and the two ways are pop and directly accessing the index
pop
The pop command removes and loads the end of the list.
For example:
my_list = [2, 4, 6, 8]
x = my_list.pop()
print(x)
#Output
8
Direct access
Just like when writing to the list you can do the same thing when reading
For example:
my_list = [1, 2, 3, 4]
x = my_list[1]
print(x)
#Output
2
Other commands
There are lots of other commands you can use on lists if there is command that is not shown here comment on this page and I will add it here
- .remove(item): removes a value from the list
- len(list): returns the amount of elements in the list
- count(item): counts how many times a element is in the list
- sort(list): sorts a list in ascending order
- reverse(): reverses order of the list
Conclusion
In this blog I have covered the basics on how to use lists in python and if you have any questions feel free to comment
Related links
This is how Python Variables and Data Types work