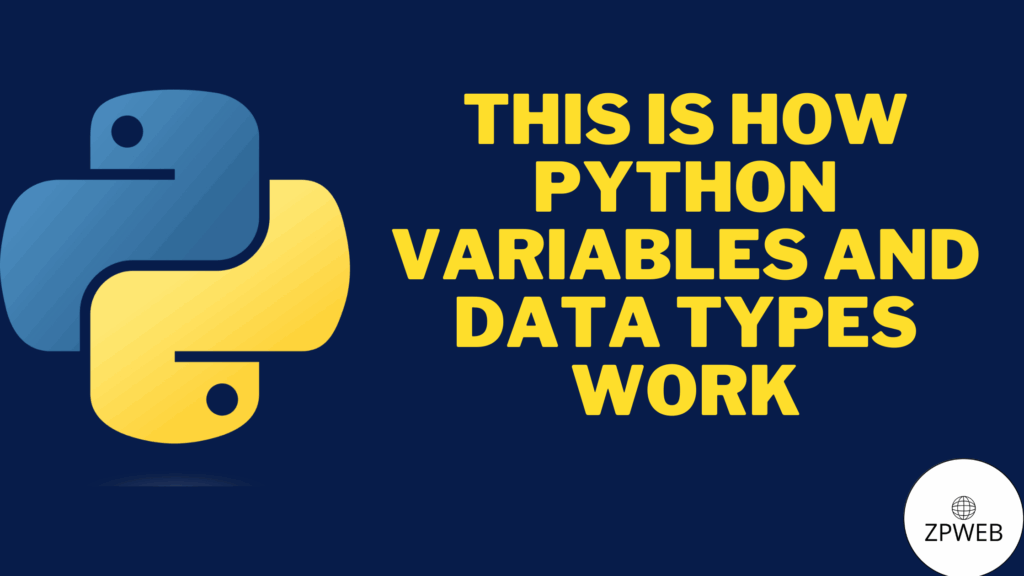
In Python functions are one of the most important parts in making anything in Python and in this posts I will share what functions are and how to use them properly and basic practices that will make your code more readable.
What are Functions
Functions in Python are just parts of the code that can be reused again by calling it. Functions in Python are useful because using functions allows you to save time and even some space on the computer because you only need to write the same code once then you can call it again whenever you want without having to completely duplicate your code and here is an example of how functions allow you to save time:
Without functions:
# Student 1
name1 = "Alice"
grade1_1 = 85
grade1_2 = 90
grade1_3 = 78
avg1 = (grade1_1 + grade1_2 + grade1_3) / 3
if avg1 >= 60:
print(f"{name1} passed with average {avg1}")
else:
print(f"{name1} failed with average {avg1}")
# Student 2
name2 = "Bob"
grade2_1 = 45
grade2_2 = 60
grade2_3 = 58
avg2 = (grade2_1 + grade2_2 + grade2_3) / 3
if avg2 >= 60:
print(f"{name2} passed with average {avg2}")
else:
print(f"{name2} failed with average {avg2}")
# Student 3
name3 = "Charlie"
grade3_1 = 92
grade3_2 = 87
grade3_3 = 85
avg3 = (grade3_1 + grade3_2 + grade3_3) / 3
if avg3 >= 60:
print(f"{name3} passed with average {avg3}")
else:
print(f"{name3} failed with average {avg3}")
With functions:
def average(grades):
return sum(grades) / len(grades)
def report_result(name, grades):
avg = average(grades)
status = "passed" if avg >= 60 else "failed"
print(f"{name} {status} with average {avg}")
# Using functions
report_result("Alice", [85, 90, 78])
report_result("Bob", [45, 60, 58])
report_result("Charlie", [92, 87, 85])
Now as you can see the code has been shortened significantly and it is also easier to read and type so now lets learn how to write a function yourself.
How to write a function
Writing a function in Python is a relatively simple process and here is how you do it.
Step 1: define the function
Whenever you write a function you declare that it is a function by using def and then give it a name and here is an example of what it looks like.
#declaring a function called function1
def function1():
Step 2: define the parameters
As you can see when I made that function you might be wondering why there are parenthesis in this function but the reason these are here is so you can put your own parameters and parameters are just variables or anything that you give a function and here is an example of how to add parameters.
#Adding parameter1 and parameter2
def function1(parameter1, parameter2):
Step 3: Implementing the function
In the code above we made a function that takes in two parameters called parameter1 and parameter2 so in this step we will be making the function do something to these variables and make an output so in this example we are going to make this function put both of these parameters in a string then put them together and print out the result and also make sure to remember to indent your code whenever you make a function, if statement or conditional and you can indent just by pressing space 4 times or pressing tab.
#Declaring the function
def function1(parameter1, parameter2):
#Doing the operation
output = f"{parameter1}{parameter2}"
#printing the output
print(output)
Step 4: Using the function
Once we have finished step 3 we have fully made the function and all that is left is using it so here is an example of the function being called.
#Declaring the function
def function1(parameter1, parameter2):
#Doing the operation
output = f"{parameter1}{parameter2}"
#printing the output
print(output)
word_prefix = "un"
words = ["able", "wrap", "safe", "seal", "real", "just"]
for word in words:
function1(word_prefix, word)
#output
unable
unwrap
unsafe
unseal
unreal
unjust
So as you can see this function just takes a list of words and adds the prefix “un” to them but you may think this is inefficient and it is but this is only an example and just like the first example I showed you there are many cases where it is actually better to not use functions but still functions are a great tool to help reduce the amount of repeated lines of code in your text and make you able to make the same code for less time and even make it easier for you to debug your code later.
Other ways to use Functions
While the first function we covered covers most of the basics and could carry you along most of your coding journey there are other cases where this basic function may not be the best solution for you so here are other ways you can use functions in your code.
Self-Referential/Recursive Functions
Recursive functions in Python are just functions that call themselves and while this may just seem like it does not have many uses but Recursive functions actually have a lot of real world uses when you need to do the same function many times and you may ask how this is different than just a for or a while loop there is actually different because those are iterators and you might again ask how iterations are different from recursions but the difference is that iterations are when you do the same thing multiple times like how multiplication is just iterated addition but recursion is when a function calls itself and here is an example of what recursion is:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
Here is an example of a recursive function and as you might notice the function calls itself recursively until it reaches an n of 0 and this function actually models the factorial function which is normally a number being multiplied by all the integers lower than it so for example the factorial of 5 would be 5x4x3x2x1 and as you can tell this function exactly models this and lets show how this function works.
factorial_num = 3
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
print(factorial(factorial_num))
Here is an example code doing the factorial of 3 so lets see what happens when you run the code and here we will not declare the function since we are just seeing inside this function.
#First Recursion
# n is 3
if n == 0: # is not 0 so skipped
return 1
else:
return n * factorial(n-1) # So here we will now be going inside of this function now
#Second Recursion
n = 2
if n == 0: # again is not 0 so skipped
return 1
else:
return n * factorial(n-1) # And yet again we are doing another recursion
#Third Recursion
n = 1
if n == 0: # I don't think I need to say this again
return 1
else:
return n * factorial(n-1) # And yet again another recursion but this time it will be the last
# Fourth Recursion
n = 1
if n == 0: # Now this is finally returning 1
return 1
else:
return n * factorial(n-1)
# So now what this does is the first recursion is 3 and it is multiplied by the second recursion multiplied by the third recursion and another one for the last but it is useless because it is still 1 so it would be like 3 * (2*1*1) which is 3*2*1 that is exactly what this function does and if you want a simple explanation it is just that there are 3 recursion layers and we just multiplied them together and every time you go down one level the number reduces by one
So now that you know how Recursive Functions work now lets do another type of function.
Dependent Functions
As the name implies dependent functions depend on other functions and here is an example of how you might use Dependent functions.
#independent function that gets country gdp
def gdp(param1):
#List of countries gdp
countries_gdp = {"USA": "$27.721 trillion", "China" : "$17.795 trillion", "Germany" : "$4.526 trillion"}
#Checks if input is in list
if param1 not in countries_gdp:
print("Country not added or recognized")
#Outputs GDP
print(countries_gdp.get(param1))
#Main dependent function that gets input
def main_func():
input_var = input("Type in Country name for GDP: ")
#Calling gdp function with input as country
gdp(input_var)
#Starts the program
main_func()
As you can see the main_func() function is totally dependent on the gdp() function for it to work so it is called a dependent function and dependent functions like these are useful when you are trying make more complex programs and you can reuse the same code again which saves your time and also makes your code more readable because you only have to debug one set of code instead of all the duplicates.
Conclusion
So now we covered most of the things you need to know about functions in python if you are still confused on some parts then you can check the sources below for further clarification and if you want to learn more and support us please read more of our blog posts and thanks for reading this blog all the way through.