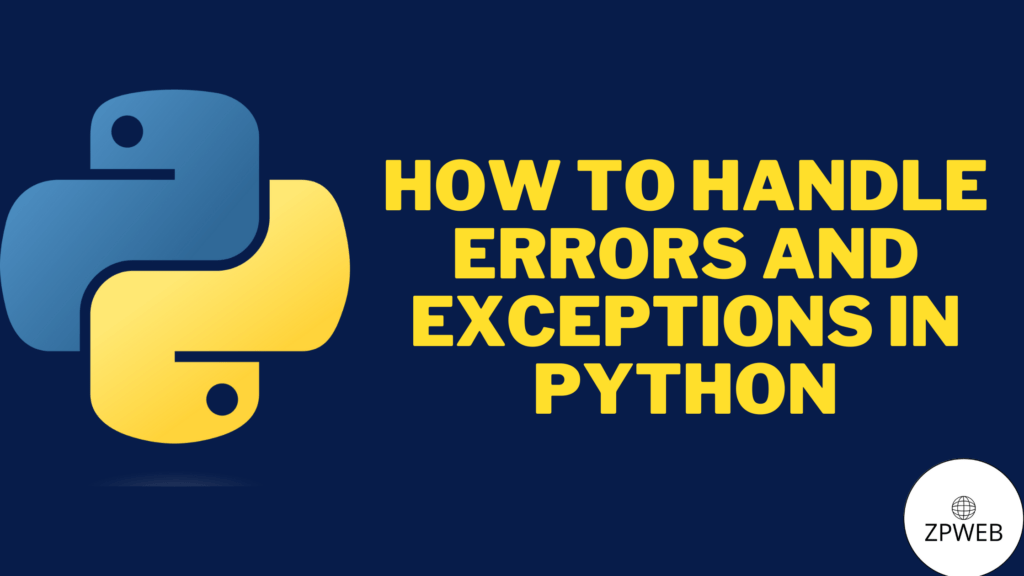
As a Python developer, it’s inevitable that you’ll encounter errors and exceptions in your code. Errors occur when the interpreter can’t understand your code, while exceptions occur during the execution of your code. Fortunately, Python has built-in mechanisms for handling these errors and exceptions.
In this blog post, we’ll cover the basics of error handling in Python, including the try-except block, raising and catching exceptions, and logging errors.
Headings:
- Understanding Errors and Exceptions in Python
- The try-except Block
- Handling Specific Exceptions
- Raising Exceptions
- Catching and Handling Exceptions
- Logging Errors
Understanding Errors and Exceptions in Python
Errors and exceptions are two different things in Python. Errors occur when the interpreter can’t understand your code, while exceptions occur during the execution of your code. When an error occurs, Python displays an error message and stops executing the program. However, with exceptions, Python provides us with a way to handle errors gracefully and continue the execution of the program.
The try-except Block
The try-except block is a fundamental concept in error handling in Python. We use the try block to place the code that may cause an exception. If an exception is raised, the except block catches it and handles it accordingly.
Handling Specific Exceptions
You can also specify which exception to catch using the except clause. This way, you can handle different exceptions in different ways.
Raising Exceptions
Sometimes, you may want to raise your own exception to indicate that something went wrong. Python allows you to raise exceptions using the raise statement.
Catching and Handling Exceptions
In addition to the try-except block, Python provides us with other constructs for handling exceptions. For example, we can use the finally block to ensure that a certain block of code always gets executed, whether an exception is raised or not.
Logging Errors
Finally, it’s always a good practice to log errors. Python provides us with a built-in logging module for this purpose.
Conclusion
In this post, we’ve covered the basics of error handling in Python, including the try-except block, raising and catching exceptions, and logging errors. As you get better at python, it’s important to remember how important good error-handling practices are.
Related links
- Python This is how Python Variables and Data Types work blog post
- Python Unlock the Magic of Python’s Object-Oriented Programming blog post
External links
- Python documentation on errors and exceptions: https://docs.python.org/3/tutorial/errors.html
- Python logging module documentation: https://docs.python.org/3/library/logging.html
Sample code:
try:
num1 = int(input("Enter a number: "))
num2 = int(input("Enter another number: "))
result = num1 / num2
print("Result: ", result)
except ValueError:
print("Invalid input, please enter a valid character.")
except ZeroDivisionError:
print("Cannot divide by zero.")
except Exception as e:
print("An error occurred: ", e)
finally:
print("Program completed.")
We hope this post has been helpful in understanding how to handle errors and exceptions in Python. Remember to always test your code thoroughly and handle errors carefully. Happy coding! For more posts click here