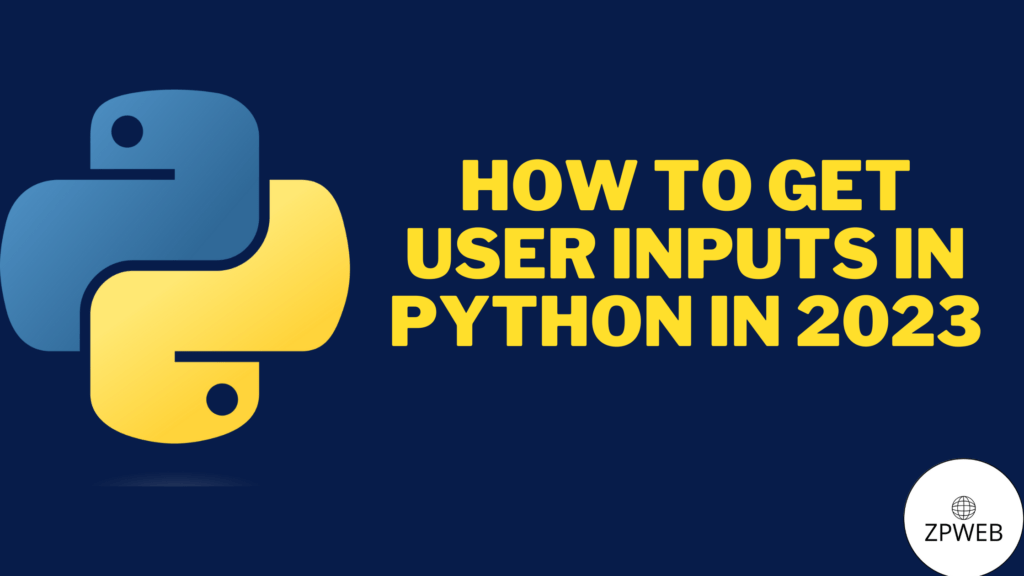
In python getting user inputs is very important since without it you will always have to manually put in variables so in this blog you will be learning how to get user inputs.
Input command
To be able to use user inputs you must use the input() command the input command will give what the user types in
example:
You can also make it say something before requesting an input
in = input()
print(in)
This will print whatever you type in console, for example, if I type ‘Hello’ it will print ‘Hello’
example:
Prompt
in = input("Enter input: ")
print(in)
The program will say ‘Enter input: ‘ and when you type the input it will print your output
You can also make the input have a default value
example:
in = input("Enter input: ", "None")
print(in)
So what the code does now is that if the user inputs nothing it will print None you can also edit the default by changing the value so if the string “None” was changed to “Nothing” then it would say “Nothing” when you didn’t type anything.
Change input variable type
You can also make it convert the user input to certain types of variables. By default the input command turns all user input into a string but you can change it by changing the type of the input
example:
in_num = int(input("Enter first number: "))
in_num2 = int(input("Enter second number: "))
print(f" {in_num} + {in_num2} is {in_num + in_num2}")
In this program it will convert the use inputs into integers so they can be added together
Conclusion
So in conclusion the input() command is very useful when you want to get user input. If you want to learn further check the extra links below